## 单例的实现多种方式 1. 绑定类 ```python """ Author:clever-cat time :2022/11/9-14:33 """ # 1.第一种,类中调用绑定给类的函数实现 class MyClass: isinstan = None def __init__(self, name, age): self.name = name self.age = age @classmethod def sigleton(cls): if not cls.isinstan: cls.isinstan = cls('张三', '李四') return cls.isinstan return cls.isinstan obj1 = MyClass('ja',1) obj2 = MyClass(12,1323) obj3 = MyClass(123,132) obj4 = MyClass(123123,123) obj5 = MyClass(123,12) # obj1 = MyClass.sigleton() # obj2 = MyClass.sigleton() # obj3 = MyClass.sigleton() # obj4 = MyClass.sigleton() # obj5 = MyClass.sigleton() print(obj1) print(obj2) print(obj3) print(obj4) print(obj5) print(MyClass.__dict__) ``` 2. 元类 ```python """ Author:clever-cat time :2022/11/9-14:46 """ # 2.元类定义模式 class MyType(type): def __init__(cls, *args, **kwargs): # 2 print('222') if not hasattr(cls, 'isinstance'): print('3333') cls.isinstance = cls.__new__(cls) cls.isinstance.__init__('json', 18) super(MyType, cls).__init__(*args,**kwargs) def __call__(cls, *args, **kwargs): if args or kwargs: print('5555') obj = cls.__new__(cls) obj.__init__(*args, **kwargs) return obj print('444') return cls.isinstance class Stundent(metaclass=MyType): def __init__(self, name, age): print('111') self.name = name self.age = age obj1 = Stundent() # 1 obj2 = Stundent() # 1 obj3 = Stundent() # 1 obj4 = Stundent() # 1 obj5 = Stundent() # 1 # obj1 = Stundent('aa', 12) # obj2 = Stundent('aa1', 12) # obj3 = Stundent('aa2', 12) # obj4 = Stundent('aa3', 12) # obj5 = Stundent('aa4', 12) print(obj1) print(obj2) print(obj3) print(obj4) print(obj5) print(Stundent.__dict__, obj1.__dict__) ``` 3. 模块导入 ```python """ Author:clever-cat time :2022/11/9-15:01 """ # 3.模块类型的 class MyClass: def __init__(self, name, age): self.name = name self.age = age obj = MyClass('json', 18) ``` 4. 装饰器实现 ```python """ Author:clever-cat time :2022/11/9-15:04 """ from functools import wraps def outer(cls): cls.instance = cls('jjjj', 18) @wraps(cls) # 伪装的更像,可以正常查看名称空间 def inner(*args, **kwargs): print(args, kwargs) if args or kwargs: cls.instance = cls(*args, **kwargs) return cls.instance # if not cls.instance: # # cls.instance = cls.__new__(cls) # # cls.instance.__init__('jjjj', 18) # cls.instance = cls('jjjj', 18) # 这一句等于上面两句 return cls.instance return inner @outer class Student: dasdas = None def __init__(self, name, age): self.name = name self.age = age # obj1 = Student() # obj2 = Student() # obj3 = Student() # obj4 = Student() # obj5 = Student() obj1 = Student('asd', 1213466233) obj2 = Student('asdasd', 3464346) obj3 = Student('asdasd', 1123231231434345123323) obj4 = Student('asdasd', 1116346461212232323) obj5 = Student('asdasd', 1122343432323) print(obj1) print(obj2) print(obj3) print(obj4) print(obj5) print(obj1.__dict__, Student.dasdas) ``` ## pickle对象序列化模块 1. 优点:能够序列化python中所有的类型 2. 缺点:只能够在python中使用 无法跨语言传输 3. 需求:产生一个对象并保存到文件中 取出来,只要存进去的类还在,在那个文件都能读 ```python class C1: def __init__(self, name, age): self.name = name self.age = age def func1(self): print('from func1') def func2(self): print('from func2') obj = C1('jason', 18) # import json # with open(r'a.txt','w',encoding='utf8') as f: # json.dump(obj, f) # import pickle # with open(r'a.txt', 'wb') as f: # pickle.dump(obj, f) # with open(r'a.txt','rb') as f: # data = pickle.load(f) # print(data) # data.func1() # data.func2() # print(data.name) ``` ## 选课系统需求分析 ```python 选课系统 角色:学校、学员、课程、讲师 要求: 1. 创建北京、上海 2 所学校 2. 创建linux , python , go 3个课程 , linux\py 在北京开, go 在上海开 3. 课程包含,周期,价格,通过学校创建课程 4. 通过学校创建班级, 班级关联课程、讲师5. 创建学员时,选择学校,关联班级 5. 创建讲师角色时要关联学校, 6. 提供三个角色接口 6.1 学员视图, 可以注册, 交学费, 选择班级, 6.2 讲师视图, 讲师可管理自己的班级, 上课时选择班级, 查看班级学员列表 , 修改所管理的学员的成绩 6.3 管理视图,创建讲师, 创建班级,创建课程 7. 上面的操作产生的数据都通过pickle序列化保存到文件里 ``` ## 功能提炼 ```python 1.管理员功能 注册功能 登录功能 创建学校 创建课程 创建老师 2.讲师功能 登录功能 选择课程 查看课程 查看学生分数 修改学生分数 3.学生功能 注册功能 登录功能 选择学校 选择课程 查看课程分数 ``` 选课系统架构设计 ```python 三层架构 1.视图层 2.业务成 3.数据处理层 3.1moudles模块产生各种方法只能由这个模块调用db_handler模块 3.2db_handler模块保存文件 ``` 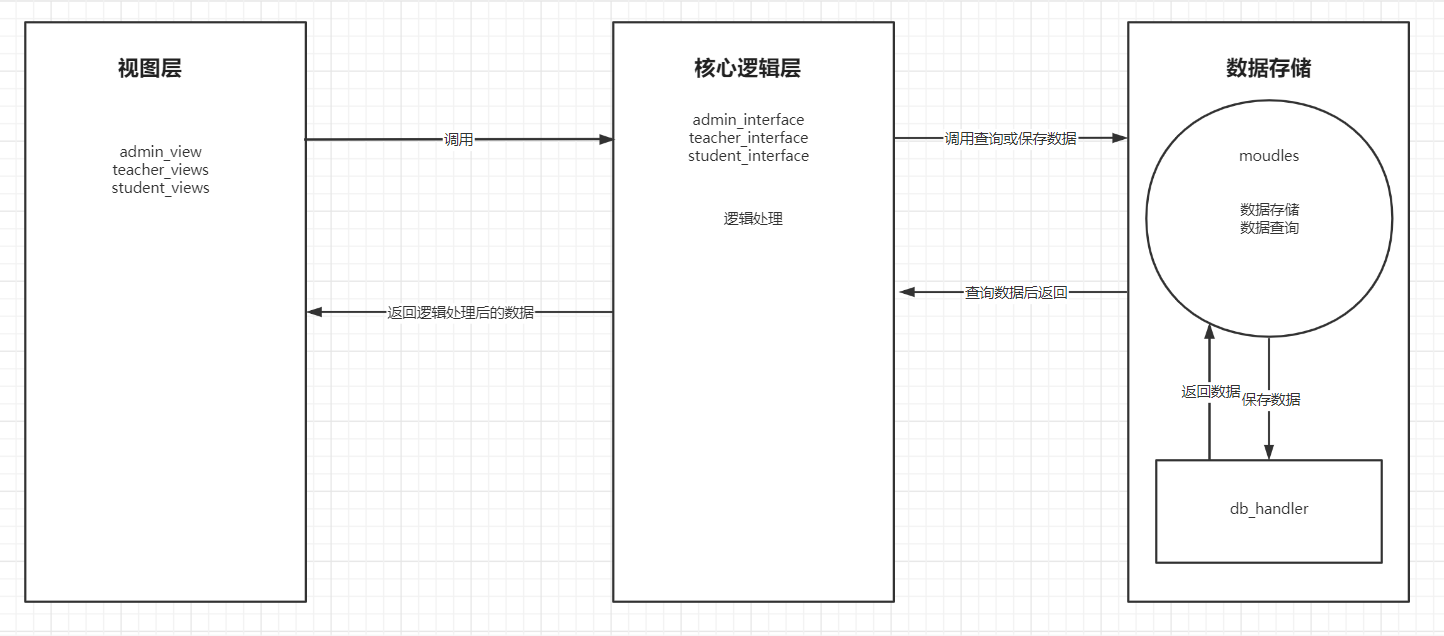 Last modification:November 9th, 2022 at 07:21 pm © 允许规范转载 Support 如果觉得我的文章对你有用,请随意赞赏 ×Close Appreciate the author Sweeping payments
Comment here is closed